728x90
반응형
[예제1]
using System;
using static System.Console;
using System.Linq;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
using System.Collections.Generic;
using System.Threading;
using System.Threading.Tasks;
namespace ConsoleApp1
{
class Program
{
// 소수 판단 프로그램이다.
public static bool IsPrime(long num)
{
if (num < 2) { return false; }
if (num % 2 == 0 && num != 2)
{
return false;
}
for (int i = 2; i < num; i++)
{
if (num % i == 0)
{ return false; }
}
return true;
}
static void Main(string[] args)
{
for (int ct = 1; ct <= 16; ct++)
{
long from = 0;
long to = 20000;
int taskCount = ct * 2;
Func<object, List<long>> FindPrimeFunc = (objRange) =>
{
long[] range = (long[])objRange;
List<long> found = new List<long>();
for (long i = range[0]; i < range[1]; i++)
{
if (IsPrime(i))
found.Add(i);
}
return found;
};
Task<List<long>>[] tasks = new Task<List<long>>[taskCount];
long currentFrom = from;
long currentTo = to / tasks.Length;
for (int i = 0; i < tasks.Length; i++)
{
tasks[i] = new Task<List<long>>(
FindPrimeFunc,
new long[] { currentFrom, currentTo }
);
//WriteLine("{0} {1}", currentFrom, currentTo);
currentFrom = currentTo + 1;
if (i == tasks.Length - 2)
currentTo = to;
else
currentTo = currentTo + (to / tasks.Length);
}
// Ready Go !!!!
//ReadLine(); // 블로킹
//WriteLine("Start !!!");
DateTime startTime = DateTime.Now;
//{
//WriteLine(startTime);
foreach (Task<List<long>> task in tasks)
task.Start();
List<long> total = new List<long>();
foreach (Task<List<long>> task in tasks)
{
task.Wait();
total.AddRange(task.Result.ToArray());
}
//}
DateTime endTime = DateTime.Now;
TimeSpan ellaped = endTime - startTime;
WriteLine("{0} {1} {2}", ct, ellaped, total.Count);
//WriteLine();
}
}
}
}
/* for (int i = 0; i < 20; i++)
{
WriteLine(i + " " + IsPrime(i));
}*/
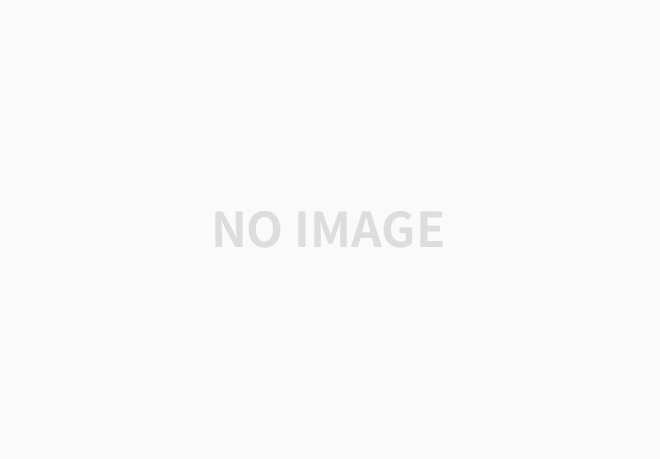
728x90
반응형
'Education > Edu | .net' 카테고리의 다른 글
# 32) [C#] Winform 2 (0) | 2021.03.02 |
---|---|
# 31) [C#] Winform 1 (0) | 2021.02.26 |
# 29.2) [C#] 문법12 (0) | 2021.02.24 |
# 29.1) [C#] 문법12 (delegate) (0) | 2021.02.24 |
# 28.2) [C#] 문법11 (Generic) (0) | 2021.02.23 |